BPMN Event in Blazor Diagram Component
30 Jun 202510 minutes to read
An Event is a common BPMN process model element that represents something that happens during a business process and is notated with a circle. The type of events are as follows:
- Start - Indicates the beginning of the process and every business process start with an event.
- Intermediate - Indicates the middle of the process.
- End - Indicates the end of the process, and every business process ends with an event.
The EventType property of the node allows you to define the type of the event. The default value of the event is Start. The following code example explains how to create a BPMN event.
@using Syncfusion.Blazor.Diagram
@* Initialize Diagram *@
<SfDiagramComponent Height="600px" Nodes="@nodes" />
@code
{
// Initialize node collection with Node.
DiagramObjectCollection<Node> nodes;
protected override void OnInitialized()
{
nodes = new DiagramObjectCollection<Node>();
Node node = new Node()
{
// Position of the node.
OffsetX = 100,
OffsetY = 100,
// Size of the node.
Width = 100,
Height = 100,
// Unique Id of the node.
ID = "node1",
//Sets shape as BpmnEvent.
Shape = new BpmnEvent()
{
// Set the event type as End.
EventType = BpmnEventType.End,
}
};
nodes.Add(node);
}
}
You can download a complete working sample from GitHub
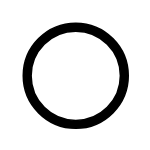
How to Create a BPMN Event Trigger
Event triggers are notated as icons inside the circle and they represent the specific details of the process. The Trigger property of the node allows you to set the type of trigger and by default, it is set to None. The following code example explains how to create a BPMN trigger.
@using Syncfusion.Blazor.Diagram
@* Initialize Diagram *@
<SfDiagramComponent Height="600px" Nodes="@nodes" />
@code
{
// Initialize node collection with Node.
DiagramObjectCollection<Node> nodes;
protected override void OnInitialized()
{
nodes = new DiagramObjectCollection<Node>();
Node node = new Node()
{
// Position of the node.
OffsetX = 100,
OffsetY = 100,
// Size of the node.
Width = 100,
Height = 100,
// Unique Id of the node.
ID = "node1",
//Sets type as Bpmn and shape as Event
Shape = new BpmnEvent()
{
// Set the event type to NonInterruptingIntermediate and set the trigger as message.
EventType = BpmnEventType.Start,
Trigger = BpmnEventTrigger.Message
}
};
nodes.Add(node);
}
}
You can download a complete working sample from GitHub
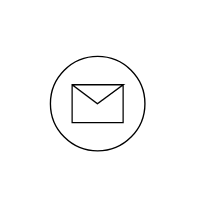
The following table illustrates the type of event triggers.
Triggers |
Start |
Non-Interrupting Start |
Intermediate |
Non-Interrupting Intermediate |
Throwing Intermediate |
End |
None |
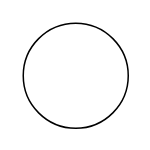 |
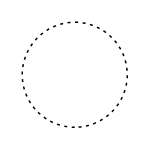 |
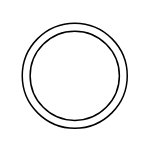 |
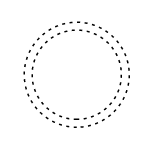 |
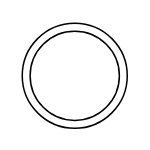 |
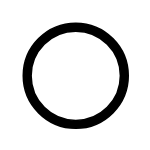 |