Disabled Items in Blazor Mention Component
17 Sep 20245 minutes to read
The Mention provides options for individual items to be either in an enabled or disabled state for specific scenarios. The category of each list item can be mapped through the Disabled field in the data table. Once an item is disabled, it cannot be selected as a value for the component. To configure the disabled item columns, use the MentionFieldSettings.Disabled
property.
In the following sample, State are grouped according on its category using Disabled
field.
@using Syncfusion.Blazor.DropDowns
<div id="mention-controls">
<table>
<tr>
<td>
<SfMention TItem="Person" DataSource="@People" PopupHeight="250px">
<TargetComponent>
<div id="commentsMention" aria-label="Mention Target" placeHolder="Type @@ and tag user" ></div>
</TargetComponent>
<ChildContent>
<MentionFieldSettings Text="Name" Disabled="State"></MentionFieldSettings>
</ChildContent>
</SfMention>
</td>
</tr>
</table>
</div>
<style>
#commentsMention {
min-height: 100px;
border: 1px solid #D7D7D7;
border-radius: 4px;
padding: 8px;
font-size: 14px;
width: 600px;
}
#mention-controls {
margin: 0 auto;
width: 600px;
}
div#commentsMention[placeholder]:empty:before {
content: attr(placeholder);
color: #555;
}
@@media screen and (max-width: 1010px) {
#mention-controls, #mention-controls table{
width: 100%;
}
}
</style>
@code {
public class Person
{
public string Name { get; set; }
public string Email { get; set; }
public string EmployeeImage { get; set; }
public bool State { get; set; }
}
private List<Person> People = new List<Person> {
new Person() { Name="Selma Rose", EmployeeImage="3", Email="[email protected]", State=true },
new Person() { Name="Russo Kay", EmployeeImage="8", Email="[email protected]", State=false },
new Person() { Name="Camden Kate", EmployeeImage="9", Email="[email protected]", State=false },
new Person() { Name="Mary Kate", EmployeeImage="4", Email="[email protected]", State=true },
new Person() { Name="Ursula Ann", EmployeeImage="2", Email="[email protected]", State=false },
new Person() { Name="Margaret", EmployeeImage="5", Email="[email protected]", State=false },
new Person() { Name="Laura Grace", EmployeeImage="6", Email="[email protected]", State=false },
new Person() { Name="Robert", EmployeeImage="8", Email="[email protected]", State=true },
new Person() { Name="Albert", EmployeeImage="9", Email="[email protected]", State=false },
new Person() { Name="Michale", EmployeeImage="10", Email="[email protected]", State=false },
new Person() { Name="Andrew James", EmployeeImage="7", Email="[email protected]", State=false },
new Person() { Name="Rosalie", EmployeeImage="4", Email="[email protected]", State=false },
new Person() { Name="Stella Ruth", EmployeeImage="2", Email="[email protected]", State=true },
new Person() { Name="Richard Rose", EmployeeImage="10", Email="[email protected]", State=false },
new Person() { Name="Gabrielle", EmployeeImage="3", Email="[email protected]", State=false },
new Person() { Name="Thomas", EmployeeImage="7", Email="[email protected]", State=false },
new Person() { Name="Charles Danny", EmployeeImage="8", Email="[email protected]", State=false },
new Person() { Name="Daniel", EmployeeImage="10", Email="[email protected]", State=true },
new Person() { Name="Matthew", EmployeeImage="7", Email="[email protected]", State=false },
new Person() { Name="Donald Krish", EmployeeImage="9", Email="[email protected]", State=false },
new Person() { Name="Yohana", EmployeeImage="1", Email="[email protected]", State=true },
new Person() { Name="Kevin Paul", EmployeeImage="10", Email="[email protected]", State=false }
};
}
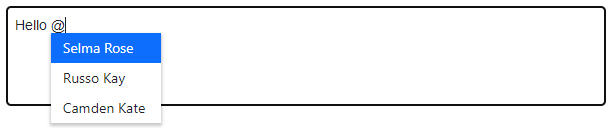
Disable Item Method
The disableItem method can be used to handle dynamic changing in disable state of a specific item. Only one item can be disabled in this method. To disable multiple items, this method can be iterated with the items list or array. The disabled field state will to be updated in the DataSource, when the item is disabled using this method.
Parameter | Type | Description |
---|---|---|
itemValue |
string | number | boolean | object
|
It accepts the string, number, boolean and object type value of the item to be removed. |
itemIndex | number |
It accepts the index of the item to be removed. |