View PDF files using PDF Viewer Component in the Blazor Web app
3 Mar 202510 minutes to read
In this section, we’ll guide you through the process of adding Syncfusion® Blazor PDF Viewer component to your Blazor Web App using Visual Studio and Visual Studio Code. We’ll break it down into simple steps to make it easy to follow.
Prerequisites
-
If you choose an Interactive render mode such as WebAssembly or Auto, ensure that you have the necessary .NET workloads installed to use the PDF Viewer component in a Blazor WebApp application with SkiaSharp. To do this, execute the following commands in the command prompt:
- dotnet workload install wasm-tools
Create a new Blazor Web App in Visual Studio
You can create a Blazor Web App using Visual Studio 2022 via Microsoft Templates or the Syncfusion® Blazor Extension.
You need to configure the corresponding Interactive render mode and Interactivity location while creating a Blazor Web Application.
Install Blazor PDF Viewer NuGet package in Blazor Web App
To add Blazor PDF Viewer component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install
If you select an Interactive render mode as WebAssembly or Auto, you can install the NuGet package in the client-side project to add component in Web App.
NOTE
On the Syncfusion® side, we are using SkiaSharp.Views.Blazor version 3.116.1. Please make sure to reference this version as well.
Prerequisites
-
If you choose an Interactive render mode such as WebAssembly or Auto, ensure that you have the necessary .NET workloads installed to use the PDF Viewer component in a Blazor WebApp application with SkiaSharp. To do this, execute the following commands in the command prompt:
- dotnet workload install wasm-tools
Create a new Blazor Web App in Visual Studio Code
You can create a Blazor Web App using Visual Studio Code via Microsoft Templates or the Syncfusion® Blazor Extension.
You need to configure the corresponding Interactive render mode and Interactivity location while creating a Blazor Web Application.
For example, in a Blazor Web App with the Auto
interactive render mode, use the following commands.
dotnet new blazor -o BlazorWebApp -int Auto
cd BlazorWebApp
cd BlazorWebApp.Client
NOTE
For more information on creating a Blazor Web App with various interactive modes and locations, refer to this link.
Install Syncfusion® Blazor SfPdfViewer and Themes NuGet in the App
If you utilize WebAssembly
or Auto
render modes in the Blazor Web App need to be install Syncfusion® Blazor components NuGet packages within the client project.
- Press Ctrl+` to open the integrated terminal in Visual Studio Code.
- Ensure you’re in the project root directory where your
.csproj
file is located. - Run the following command to install a Syncfusion.Blazor.SfPdfViewer and Syncfusion.Blazor.Themes NuGet package and ensure all dependencies are installed.
dotnet add package Syncfusion.Blazor.SfPdfViewer -v 29.1.33
dotnet add package Syncfusion.Blazor.Themes -v 29.1.33
dotnet restore
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
NOTE
On the Syncfusion® side, we are using SkiaSharp.Views.Blazor version 3.116.1. Please make sure to reference this version as well.
- dotnet add package SkiaSharp.Views.Blazor -v 3.116.1
Register Syncfusion® Blazor Service
Interactive Render Mode | Description |
---|---|
WebAssembly or Auto | Open ~/_Imports.razor file from the client project. |
Server | Open ~/_import.razor file, which is located in the Components folder. |
- In the ~/_Imports.razor file, add the following namespaces:
@using Syncfusion.Blazor;
@using Syncfusion.Blazor.SfPdfViewer;
- Register the Syncfusion® Blazor Service in the program.cs file of your Blazor Web App.
If the Interactive Render Mode is set to WebAssembly
or Auto
, you need to register the Syncfusion® Blazor service in both ~/Program.cs files of your Blazor Web App.
If the Interactive Render Mode is set to Server
, your project will contain a single ~/Program.cs file. So, you should register the Syncfusion® Blazor Service only in that ~/Program.cs file.
using BlazorWebAppServer.Components;
using Syncfusion.Blazor;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddRazorComponents()
.AddInteractiveServerComponents();
builder.Services.AddSignalR(o => { o.MaximumReceiveMessageSize = 102400000; });
builder.Services.AddMemoryCache();
//Add Syncfusion Blazor service to the container.
builder.Services.AddSyncfusionBlazor();
var app = builder.Build();
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error", createScopeForErrors: true);
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseAntiforgery();
app.MapRazorComponents<App>()
.AddInteractiveServerRenderMode();
app.Run();
using BlazorWebApp.Client.Pages;
using BlazorWebApp.Components;
using Syncfusion.Blazor;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddRazorComponents()
.AddInteractiveWebAssemblyComponents();
builder.Services.AddMemoryCache();
//Add Syncfusion Blazor service to the container
builder.Services.AddSyncfusionBlazor();
var app = builder.Build();
if (app.Environment.IsDevelopment())
{
app.UseWebAssemblyDebugging();
}
else
{
app.UseExceptionHandler("/Error", createScopeForErrors: true);
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseAntiforgery();
app.MapRazorComponents<App>()
.AddInteractiveWebAssemblyRenderMode()
.AddAdditionalAssemblies(typeof(Counter).Assembly);
app.Run();
using BlazorWebAppAuto.Client.Pages;
using BlazorWebAppAuto.Components;
using Syncfusion.Blazor;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddRazorComponents()
.AddInteractiveServerComponents() .AddInteractiveWebAssemblyComponents();
builder.Services.AddSignalR(o => { o.MaximumReceiveMessageSize = 102400000; });
builder.Services.AddMemoryCache();
//Add Syncfusion Blazor service to the container
builder.Services.AddSyncfusionBlazor();
var app = builder.Build();
if (app.Environment.IsDevelopment())
{
app.UseWebAssemblyDebugging();
}
else
{ app.UseExceptionHandler("/Error", createScopeForErrors: true);
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseAntiforgery();
app.MapRazorComponents<App>()
.AddInteractiveServerRenderMode() .AddInteractiveWebAssemblyRenderMode()
.AddAdditionalAssemblies(typeof(Counter).Assembly);
NOTE
Processing Large Files Without Increasing Maximum Message Size in SfPdfViewer Component
Adding stylesheet and script
Add the following stylesheet and script to the head section of the ~/Components/App.razor file.
<head>
<!-- Syncfusion Blazor PDF Viewer control's theme style sheet -->
<link href="_content/Syncfusion.Blazor.Themes/bootstrap5.css" rel="stylesheet" />
<!-- Syncfusion Blazor PDF Viewer control's scripts -->
<script src="_content/Syncfusion.Blazor.SfPdfViewer/scripts/syncfusion-blazor-sfpdfviewer.min.js" type="text/javascript"></script>
</head>
Adding Blazor PDF Viewer Component
Add the Syncfusion® PDF Viewer (Next Gen) component in the ~Pages/.razor file. If an interactivity location as Per page/component
in the web app, define a render mode at the top of the ~Pages/.razor component, as follows:
Interactivity location | RenderMode | Code |
---|---|---|
Per page/component | Auto | @rendermode InteractiveAuto |
WebAssembly | @rendermode InteractiveWebAssembly | |
Server | @rendermode InteractiveServer | |
None | — |
NOTE
If an Interactivity Location is set to
Global
and the Render Mode is set toAuto
orWebAssembly
orServer
, the render mode is configured in theApp.razor
file by default.
@* Your App render mode define here *@
@rendermode InteractiveAuto
NOTE
If an interactivity location as Global no need to mention render mode. Set the interactivity mode for whole sample.
Add the Syncfusion® PDF Viewer component in the ~/Pages/Index.razor file.
@page "/"
<SfPdfViewer2 DocumentPath="https://cdn.syncfusion.com/content/pdf/pdf-succinctly.pdf"
Height="100%"
Width="100%">
</SfPdfViewer2>
NOTE
If you don’t provide the DocumentPath property value, the PDF Viewer component will be rendered without loading the PDF document. Users can then use the open option from the toolbar to browse and open the PDF as required.
Run the application
Run the application, and the PDF file will be displayed using Syncfusion® Blazor PDF Viewer component in your browser.
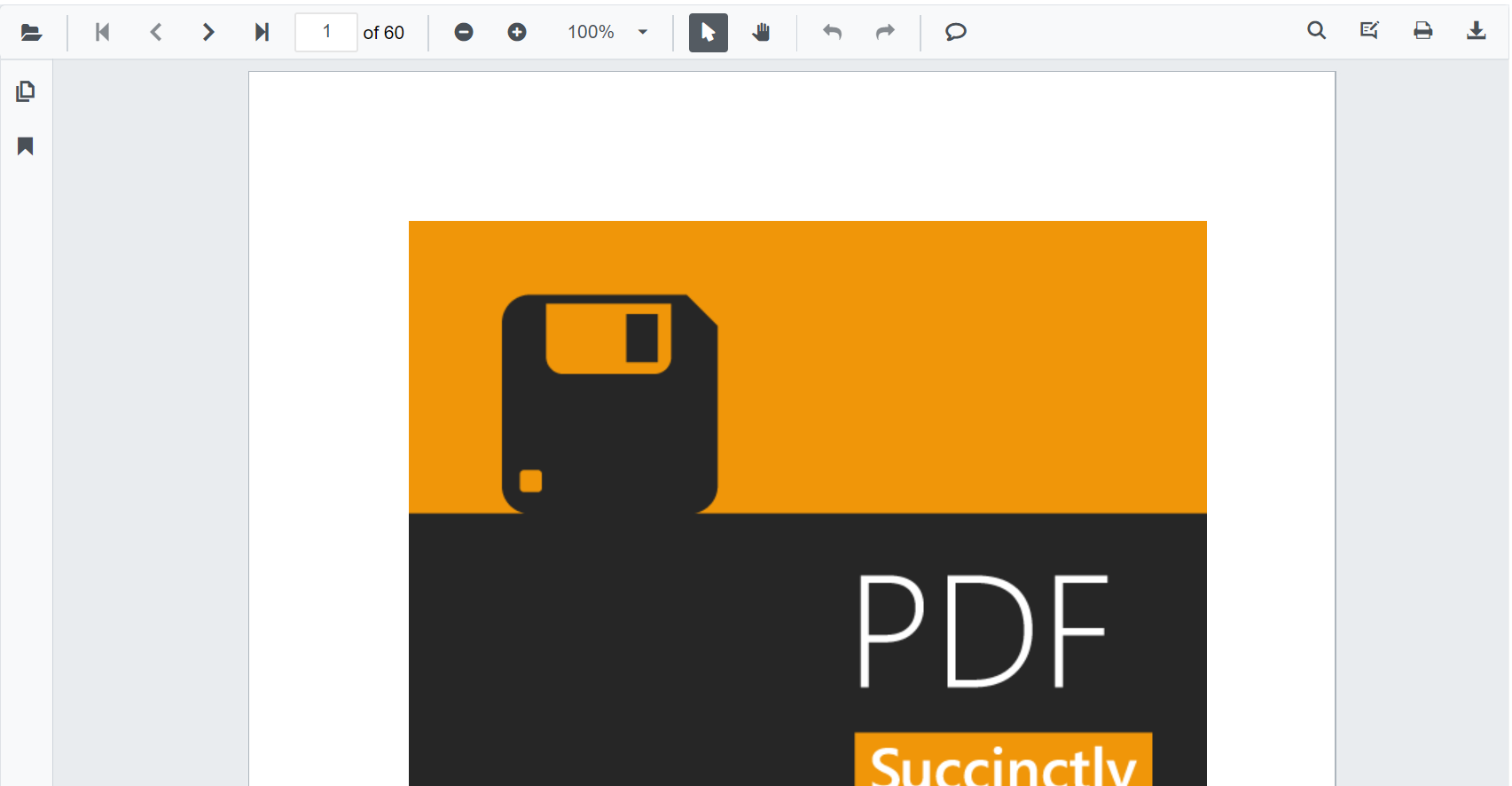
NOTE
See also
-
Getting Started with Blazor PDF Viewer Component in Blazor WASM App
-
Getting Started with Blazor PDF Viewer Component in WSL mode
-
Learn different ways to add script reference in Blazor Application
-
Processing Large Files Without Increasing Maximum Message Size in SfPdfViewer Component
-
.NET 9 Native Linking Issues with SkiaSharp and Emscripten 3.1.56