Getting Started with Blazor Barcode Component
28 Feb 20255 minutes to read
This section briefly explains about how to include Blazor Barcode component in your Blazor WebAssembly App using Visual Studio and Visual Studio Code.
Prerequisites
Create a new Blazor App in Visual Studio
You can create a Blazor WebAssembly App using Visual Studio via Microsoft Templates or the Syncfusion® Blazor Extension.
Install Syncfusion® Blazor BarcodeGenerator and Themes NuGet in the App
To add Blazor Barcode component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.BarcodeGenerator and Syncfusion.Blazor.Themes. Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.BarcodeGenerator -Version 29.1.33
Install-Package Syncfusion.Blazor.Themes -Version 29.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Prerequisites
Create a new Blazor App in Visual Studio Code
You can create a Blazor WebAssembly App using Visual Studio Code via Microsoft Templates or the Syncfusion® Blazor Extension.
Alternatively, you can create a WebAssembly application using the following command in the terminal(Ctrl+`).
dotnet new blazorwasm -o BlazorApp
cd BlazorApp
Install Syncfusion® Blazor BarcodeGenerator and Themes NuGet in the App
- Press Ctrl+` to open the integrated terminal in Visual Studio Code.
- Ensure you’re in the project root directory where your
.csproj
file is located. - Run the following command to install a Syncfusion.Blazor.BarcodeGenerator and Syncfusion.Blazor.Themes NuGet package and ensure all dependencies are installed.
dotnet add package Syncfusion.Blazor.BarcodeGenerator -v 29.1.33
dotnet add package Syncfusion.Blazor.Themes -v 29.1.33
dotnet restore
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor namespace.
@using Syncfusion.Blazor
@using Syncfusion.Blazor.BarcodeGenerator
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor WebAssembly App.
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Syncfusion.Blazor;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(serviceProvider => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddSyncfusionBlazor();
await builder.Build().RunAsync();
....
Add stylesheet and script resources
The theme stylesheet and script can be accessed from NuGet through Static Web Assets. Include the stylesheet and script references in the <head>
section of the ~/index.html file.
<head>
....
<link href="_content/Syncfusion.Blazor.Themes/bootstrap5.css" rel="stylesheet" />
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</head>
NOTE
Check out the Blazor Themes topic to discover various methods (Static Web Assets, CDN, and CRG) for referencing themes in your Blazor application. Also, check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Blazor Barcode component
Add the Syncfusion® Blazor Barcode component in the ~/Pages/Index.razor file.
<SfBarcodeGenerator Width="200px" Height="150px" Type="@BarcodeType.Codabar" Value="123456789"></SfBarcodeGenerator>
- Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the application. This will render the Syncfusion® Blazor Barcode component in your default web browser.
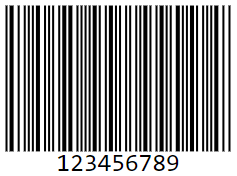
NOTE
Adding QR Generator control
You can add the QR code in our barcode generator component.
<SfQRCodeGenerator Width="200px" Height="150px" Value="Syncfusion"></SfQRCodeGenerator>
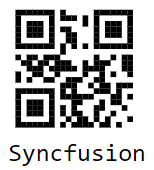
Adding Data Matrix Generator control
You can add the Data Matrix code in our barcode generator component.
<SfDataMatrixGenerator Width="200" Height="150" Value="SYNCFUSION"></SfDataMatrixGenerator>
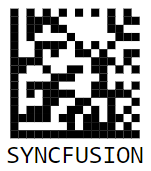
NOTE
You can also explore our Blazor Barcode Generator example that shows you how to render and configure the barcode.