Getting Started with Blazor Bullet Chart Component
28 Feb 20256 minutes to read
This section briefly explains about how to include Blazor Bullet Chart component in your Blazor WebAssembly App using Visual Studio and Visual Studio Code.
To get start quickly with Bullet Chart Component using Blazor, you can check on this video or GitHub sample:
Prerequisites
Create a new Blazor App in Visual Studio
You can create a Blazor WebAssembly App using Visual Studio via Microsoft Templates or the Syncfusion® Blazor Extension.
Install Syncfusion® Blazor Bullet Chart NuGet in the App
To add Blazor Bullet Chart component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.BulletChart. Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.BulletChart -Version 29.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Prerequisites
Create a new Blazor App in Visual Studio Code
You can create a Blazor WebAssembly App using Visual Studio Code via Microsoft Templates or the Syncfusion® Blazor Extension.
Alternatively, you can create a WebAssembly application using the following command in the terminal(Ctrl+`).
dotnet new blazorwasm -o BlazorApp
cd BlazorApp
Install Syncfusion® Blazor BulletChart and Themes NuGet in the App
- Press Ctrl+` to open the integrated terminal in Visual Studio Code.
- Ensure you’re in the project root directory where your
.csproj
file is located. - Run the following command to install a Syncfusion.Blazor.BulletChart and Syncfusion.Blazor.Themes NuGet package and ensure all dependencies are installed.
dotnet add package Syncfusion.Blazor.BulletChart -v 29.1.33
dotnet restore
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor
and Syncfusion.Blazor.Charts
namespace.
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Charts
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor WebAssembly App.
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Syncfusion.Blazor;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(serviceProvider => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddSyncfusionBlazor();
await builder.Build().RunAsync();
....
Add script resources
The script can be accessed through Static Web Assets. Include the script reference in the <head>
section of the ~/index.html file.
<head>
....
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</head>
NOTE
Check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Blazor Bullet Chart component
Add the Syncfusion® Blazor Bullet Chart component in the ~/Pages/Index.razor file.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50">
</SfBulletChart>
@code{
public class ChartData
{
public double FieldValue { get; set; }
public double TargetValue { get; set; }
}
public List<ChartData> BulletChartData = new List<ChartData>
{
new ChartData { FieldValue = 270, TargetValue = 250 }
};
}
- Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the application. This will render the Syncfusion® Blazor Bullet Chart component in your default web browser.

Adding title
Add a title by using the Title property in the Bullet Chart to provide quick information to the user about the data plotted in the component.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50" Title="Revenue">
</SfBulletChart>

Adding ranges
Add ranges by using the BulletChartRangeCollection to measure the qualitative state by observing the distance between each range.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50" Title="Revenue">
<BulletChartRangeCollection>
<BulletChartRange End=150> </BulletChartRange>
<BulletChartRange End=250></BulletChartRange>
<BulletChartRange End=300></BulletChartRange>
</BulletChartRangeCollection>
</SfBulletChart>

Adding tooltip
Use the tooltip to show the measured values by setting the Enable property to true in the BulletChartTooltip.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50" Title="Revenue">
<BulletChartTooltip TValue="ChartData" Enable="true"></BulletChartTooltip>
<BulletChartRangeCollection>
<BulletChartRange End=150> </BulletChartRange>
<BulletChartRange End=250></BulletChartRange>
<BulletChartRange End=300></BulletChartRange>
</BulletChartRangeCollection>
</SfBulletChart>
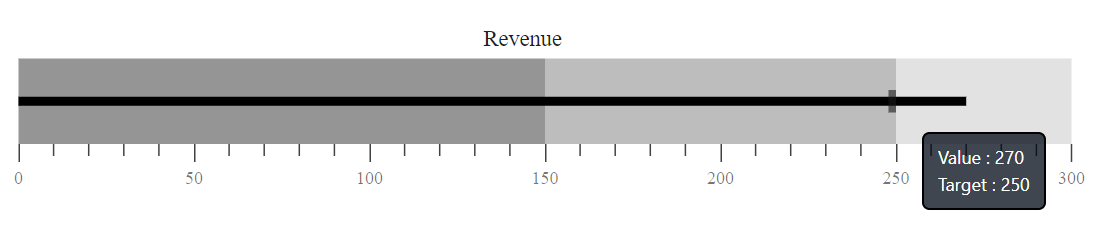
NOTE
You can also explore our Blazor Bullet Chart example that shows you how to render and configure the bullet chart.