Getting Started with Predefined Dialogs in Blazor
15 Apr 20257 minutes to read
This section briefly explains how to include the predefined dialogs in your Blazor WebAssembly App using Visual Studio and Visual Studio Code.
Prerequisites
Create a new Blazor App in Visual Studio
You can create a Blazor WebAssembly App using Visual Studio via Microsoft Templates or the Syncfusion® Blazor Extension.
Install Syncfusion® Blazor Popups and Themes NuGet in the App
To add Blazor predefined dialog component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.Popups and Syncfusion.Blazor.Themes. Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.Popups -Version 30.1.37
Install-Package Syncfusion.Blazor.Themes -Version 30.1.37
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Prerequisites
Create a new Blazor App in Visual Studio Code
You can create a Blazor WebAssembly App using Visual Studio Code via Microsoft Templates or the Syncfusion® Blazor Extension.
Alternatively, you can create a WebAssembly application using the following command in the terminal(Ctrl+`).
dotnet new blazorwasm -o BlazorApp
cd BlazorApp
Install Syncfusion® Blazor Popups and Themes NuGet in the App
- Press Ctrl+` to open the integrated terminal in Visual Studio Code.
- Ensure you’re in the project root directory where your
.csproj
file is located. - Run the following command to install a Syncfusion.Blazor.Popups and Syncfusion.Blazor.Themes NuGet package and ensure all dependencies are installed.
dotnet add package Syncfusion.Blazor.Popups -v 30.1.37
dotnet add package Syncfusion.Blazor.Themes -v 30.1.37
dotnet restore
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service and Syncfusion Dialog Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor
and Syncfusion.Blazor.Popups
namespace.
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Popups
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor WebAssembly App.
using Syncfusion.Blazor
using Syncfusion.Blazor.Popups
// Add services to the container.
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddScoped<SfDialogService>();
builder.Services.AddSyncfusionBlazor();
await builder.Build().RunAsync();
....
Add stylesheet and script resources
The theme stylesheet and script can be accessed from NuGet through Static Web Assets. Include the stylesheet and script references in the <head>
section of the ~/index.html file.
<head>
....
<link href="_content/Syncfusion.Blazor.Themes/bootstrap5.css" rel="stylesheet" />
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</head>
NOTE
Check out the Blazor Themes topic to discover various methods (Static Web Assets, CDN, and CRG) for referencing themes in your Blazor application. Also, check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Blazor Dialog Provider
SfDialogProvider
allows to open predefined dialogs based on SfDialogService
settings from any where in application. You can add SfDialogProvider
in MainLayout.razor
or any page. But it should be added only once in the app. If you add in MainLayout.razor
, you can open predefined dialogs from any where in application. If you add in particular page, you can open dialogs only within the page.
- Now, add
SfDialogProvider
in the ~/_MainLayout.razor file.
<Syncfusion.Blazor.Popups.SfDialogProvider/>
Open Predefined Dialog
Once you added SfDialogService
and SfDialogProvider
, you can open predefined dialogs from any where in application using AlertAsync,ConfirmAsync or PromptAsync methods in DialogServices.
Show alert dialog
An alert dialog box used to display an errors, warnings, and information alerts that needs user awareness. This can be achieved by using the DialogService.AlertAsync method. The alert dialog is displayed along with the OK
button. When user clicks on OK
button, alert dialog will get closed.
In the code example below, an alert dialog is displayed upon clicking the button using the Syncfusion® Blazor Button Component.
@using Syncfusion.Blazor.Popups
@using Syncfusion.Blazor.Buttons
@inject SfDialogService DialogService
<div>
<SfButton @onclick="@AlertBtn">Alert</SfButton>
</div>
<div class="status" style ="padding-top:10px">@DialogStatus</div>
@code {
private string DialogStatus { get; set; }
private async Task AlertBtn()
{
await DialogService.AlertAsync("10% of battery remaining", "Low Battery");
this.DialogStatus = "The user closed the Alert dialog";
}
}
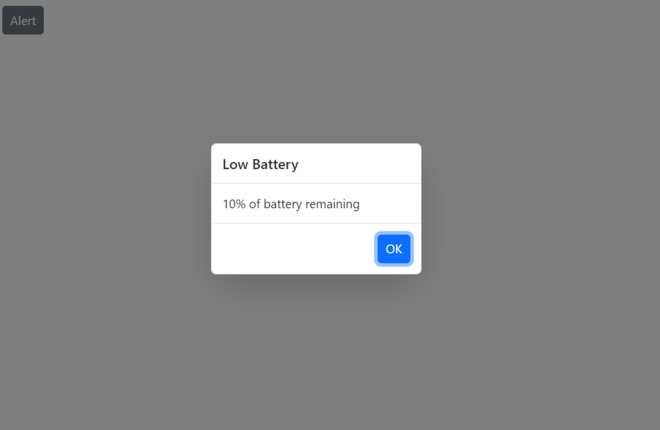
Show confirm dialog
A confirm dialog box used to displays a specified message along with the OK
and Cancel
buttons, which returns a boolean value according to the user’s click action. When the user clicks the OK
button, the true
value is returned otherwise it will returns the false
value. This can be achieved by using the DialogService.ConfirmAsync method. It is used to get approval from the user, and it appears before any critical action. After get approval from the user the dialog will disappear automatically.
In the below code example, the confirm dialog displayed on OK
and Cancel
button click action.
@using Syncfusion.Blazor.Popups
@using Syncfusion.Blazor.Buttons
@inject SfDialogService DialogService
<div>
<SfButton @onclick="@ConfirmBtn">Confirm</SfButton>
</div>
<div class="status" style ="padding-top:10px">
@DialogStatus
</div>
@code {
private string DialogStatus { get; set; }
private async Task ConfirmBtn()
{
bool isConfirm = await DialogService.ConfirmAsync("Are you sure you want to permanently delete these items?", "Delete Multiple Items");
string confirmMessage = isConfirm ? "confirmed" : "canceled";
this.DialogStatus = $"The user {confirmMessage} the dialog box.";
}
}
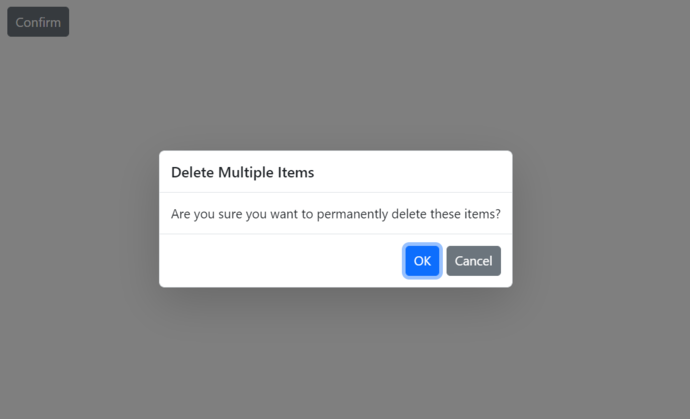
Show prompt dialog
A prompt dialog is used to get the input from the user by using the DialogService.PromptAsync method. When the user clicks the OK
button the input value from the dialog is returned. If the user clicks the Cancel
button the null
value is returned. After getting the input from the user the dialog will disappear automatically.
In the below code example, the prompt dialog displayed on OK
and Cancel
button click action.
@using Syncfusion.Blazor.Popups
@using Syncfusion.Blazor.Buttons
@inject SfDialogService DialogService
<div>
<SfButton @onclick="@PromptBtn">Prompt</SfButton>
</div>
<div class="status" style ="padding-top:10px">
@DialogStatus
</div>
@code {
private string DialogStatus { get; set; }
private async Task PromptBtn()
{
string promptText = await DialogService.PromptAsync("Enter your name:", "Join Chat Group");
this.DialogStatus = $"Input from the user is returned as \"{promptText}\".";
}
}
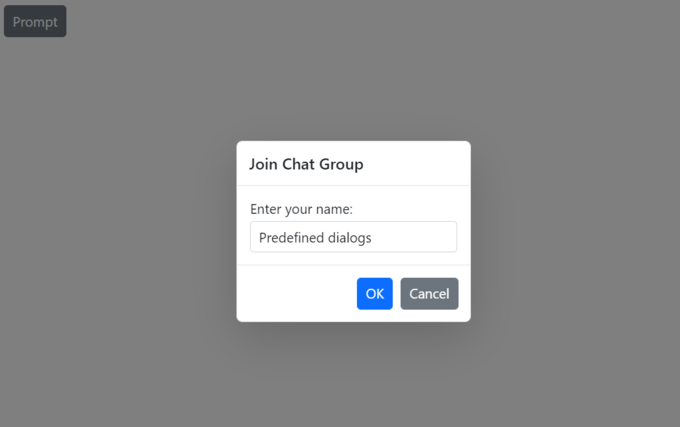