Getting Started with Blazor BulletChart in Blazor Web App
29 Nov 20245 minutes to read
This section briefly explains about how to include Blazor Bullet Chart component in your Blazor Web App using Visual Studio.
Prerequisites
Create a new Blazor Web App
You can create a Blazor Web App using Visual Studio 2022 via Microsoft Templates or the Syncfusion® Blazor Extension.
You need to configure the corresponding Interactive render mode and Interactivity location while creating a Blazor Web Application.
Install Syncfusion® Blazor Bullet Chart NuGet in the App
To add Blazor Bullet Chart component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.BulletChart.
If you utilize WebAssembly or Auto
render modes in the Blazor Web App need to be install Syncfusion® Blazor components NuGet packages within the client project.
Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.BulletChart -Version 28.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor
and Syncfusion.Blazor.Charts
namespace .
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Charts
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor Web App.
If you select an Interactive render mode as WebAssembly
or Auto
, you need to register the Syncfusion® Blazor service in both ~/Program.cs files of your Blazor Web App.
....
using Syncfusion.Blazor;
....
builder.Services.AddSyncfusionBlazor();
....
Add script resources
The script can be accessed from NuGet through Static Web Assets. Include the script reference at the end of the <body>
in the ~/Components/App.razor file as shown below:
<body>
....
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</body>
NOTE
Check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Syncfusion® Blazor Bullet Chart component
Add the Syncfusion® Blazor Bullet Chart component in the ~Pages/.razor file. If an interactivity location as Per page/component
in the web app, define a render mode at the top of the ~Pages/.razor
component, as follows:
@* desired render mode define here *@
@rendermode InteractiveAuto
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50">
</SfBulletChart>
@code{
public class ChartData
{
public double FieldValue { get; set; }
public double TargetValue { get; set; }
}
public List<ChartData> BulletChartData = new List<ChartData>
{
new ChartData { FieldValue = 270, TargetValue = 250 }
};
}
- Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the application. This will render the Syncfusion® Blazor Bullet Chart component in your default web browser.

NOTE
Adding title
Add a title by using the Title property in the Bullet Chart to provide quick information to the user about the data plotted in the component.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50" Title="Revenue">
</SfBulletChart>

Adding ranges
Add ranges by using the BulletChartRangeCollection to measure the qualitative state by observing the distance between each range.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="
0" Maximum="300" Interval="50" Title="Revenue">
<BulletChartRangeCollection>
<BulletChartRange End=150> </BulletChartRange>
<BulletChartRange End=250></BulletChartRange>
<BulletChartRange End=300></BulletChartRange>
</BulletChartRangeCollection>
</SfBulletChart>

Adding tooltip
Use the tooltip to show the measured values by setting the Enable property to true in the BulletChartTooltip.
<SfBulletChart DataSource="@BulletChartData" ValueField="FieldValue" TargetField="TargetValue" Minimum="0" Maximum="300" Interval="50" Title="Revenue">
<BulletChartTooltip TValue="ChartData" Enable="true"></BulletChartTooltip>
<BulletChartRangeCollection>
<BulletChartRange End=150> </BulletChartRange>
<BulletChartRange End=250></BulletChartRange>
<BulletChartRange End=300></BulletChartRange>
</BulletChartRangeCollection>
</SfBulletChart>
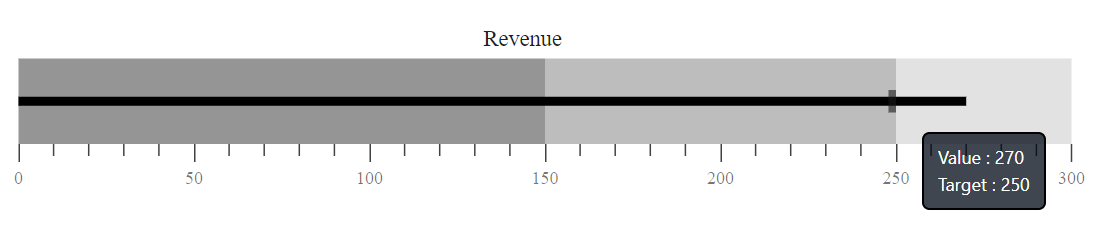
NOTE
You can also explore our Blazor Bullet Chart example that shows you how to render and configure the bullet chart.