Getting Started with Blazor Chart Component in Blazor WASM App
29 Nov 20248 minutes to read
This section briefly explains about how to include Blazor Charts component in your Blazor WebAssembly (WASM) App using Visual Studio.
Prerequisites
Create a new Blazor App in Visual Studio
You can create a Blazor WebAssembly App using Visual Studio via Microsoft Templates or the Syncfusion® Blazor Extension.
Install Syncfusion® Blazor Charts NuGet in the App
To add Blazor Chart component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.Charts. Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.Charts -Version 28.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor namespace.
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Charts
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor WebAssembly App.
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Syncfusion.Blazor;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddSyncfusionBlazor();
await builder.Build().RunAsync();
....
Add script resources
The script can be accessed from NuGet through Static Web Assets. Reference the script in the <head>
of the main page as follows:
- For Blazor WebAssembly app, include it in the ~/index.html file.
<head>
....
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</head>
NOTE
Check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Blazor Chart Component
Add the Syncfusion® Blazor Chart component in the ~/Pages/Index.razor file.
<SfChart>
</SfChart>
- Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the application. This will render the Syncfusion® Blazor Chart component in your default web browser.
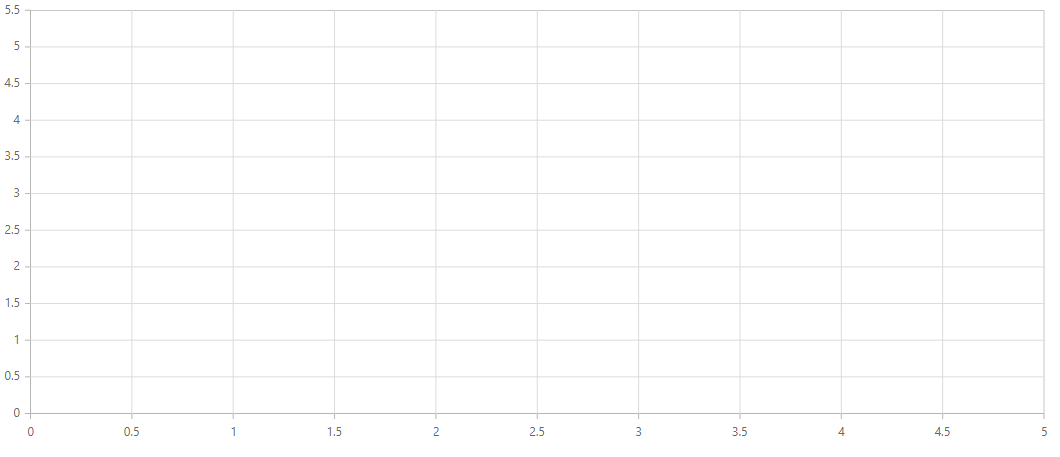
Populate Blazor chart with data
To bind data for the chart component, you can assign a IEnumerable object to the DataSource property. It can also be provided as an instance of the DataManager.
public class SalesInfo
{
public string Month { get; set; }
public double SalesValue { get; set; }
}
public List<SalesInfo> Sales = new List<SalesInfo>
{
new SalesInfo { Month = "Jan", SalesValue = 35 },
new SalesInfo { Month = "Feb", SalesValue = 28 },
new SalesInfo { Month = "Mar", SalesValue = 34 },
new SalesInfo { Month = "Apr", SalesValue = 32 },
new SalesInfo { Month = "May", SalesValue = 40 },
new SalesInfo { Month = "Jun", SalesValue = 32 },
new SalesInfo { Month = "Jul", SalesValue = 35 }
};
Now, map the data fields Month
and Sales
to the series XName and YName properties, then set the data to the DataSource property, and the chart type to Column because we will be viewing the data in a column chart.
<SfChart>
<ChartPrimaryXAxis ValueType="Syncfusion.Blazor.Charts.ValueType.Category"></ChartPrimaryXAxis>
<ChartSeriesCollection>
<ChartSeries DataSource="@Sales" XName="Month" YName="SalesValue" Type="ChartSeriesType.Column">
</ChartSeries>
</ChartSeriesCollection>
</SfChart>
@code {
public class SalesInfo
{
public string Month { get; set;}
public double SalesValue { get; set;}
}
public List<SalesInfo> Sales = new List<SalesInfo>
{
new SalesInfo { Month = "Jan", SalesValue = 35 },
new SalesInfo { Month = "Feb", SalesValue = 28 },
new SalesInfo { Month = "Mar", SalesValue = 34 },
new SalesInfo { Month = "Apr", SalesValue = 32 },
new SalesInfo { Month = "May", SalesValue = 40 },
new SalesInfo { Month = "Jun", SalesValue = 32 },
new SalesInfo { Month = "Jul", SalesValue = 35 }
};
}
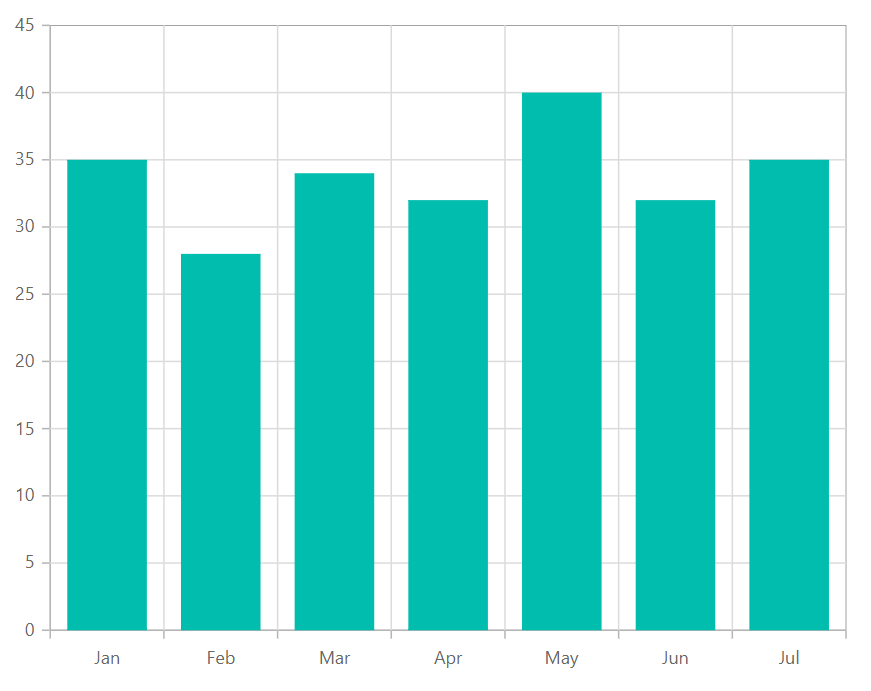
Add titles
Using the Title property, you can add a title to the chart and the axes to provide the user with quick information about the data plotted in the chart.
<SfChart Title="Sales Analysis">
<ChartPrimaryXAxis Title="Month" ValueType="Syncfusion.Blazor.Charts.ValueType.Category"></ChartPrimaryXAxis>
<ChartPrimaryYAxis Title="Sales in Dollar"></ChartPrimaryYAxis>
<ChartSeriesCollection>
<ChartSeries DataSource="@Sales" XName="Month" YName="SalesValue" Type="ChartSeriesType.Column">
</ChartSeries>
</ChartSeriesCollection>
</SfChart>
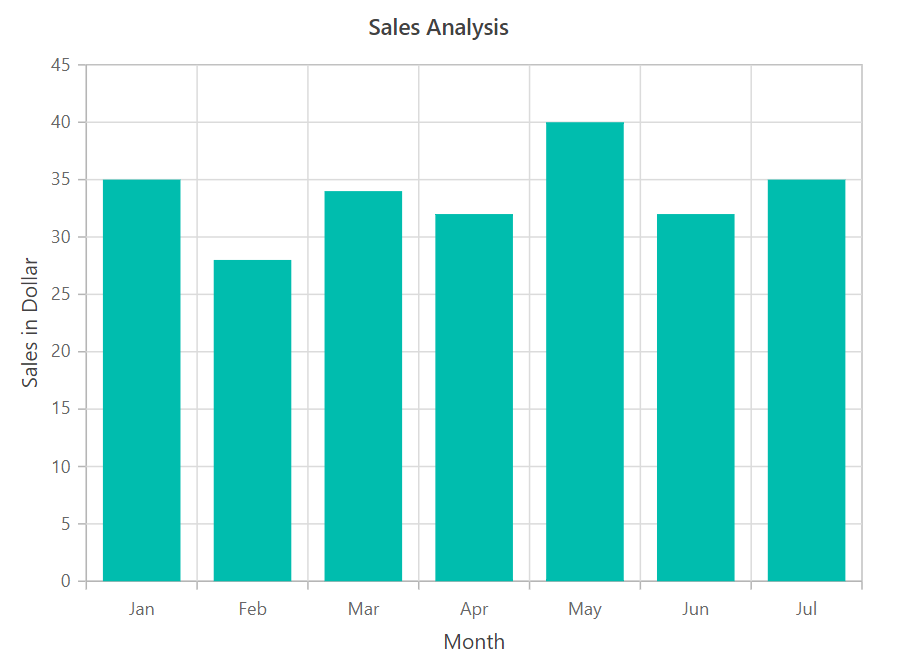
Add data label
You can add data labels to improve the readability of the chart. This can be achieved by setting the Visible property to true in the ChartDataLabel.
<SfChart Title="Sales Analysis">
<ChartPrimaryXAxis Title="Month" ValueType="Syncfusion.Blazor.Charts.ValueType.Category"></ChartPrimaryXAxis>
<ChartPrimaryYAxis Title="Sales in Dollar"></ChartPrimaryYAxis>
<ChartSeriesCollection>
<ChartSeries DataSource="@Sales" XName="Month" YName="SalesValue" Type="ChartSeriesType.Column">
<ChartMarker>
<ChartDataLabel Visible="true"></ChartDataLabel>
</ChartMarker>
</ChartSeries>
</ChartSeriesCollection>
</SfChart>
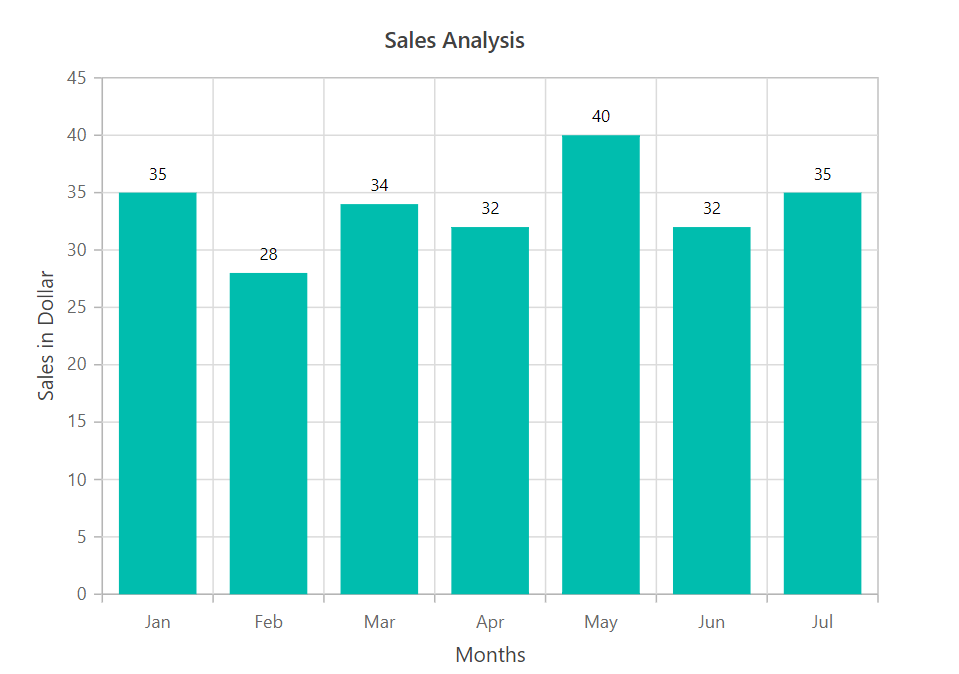
Enable Tooltip
When space constraints prevent you from displaying information using data labels, the tooltip comes in handy. The tooltip can be enabled by setting the Enable property in ChartTooltipSettings to true.
<SfChart Title="Sales Analysis">
<ChartPrimaryXAxis Title="Month" ValueType="Syncfusion.Blazor.Charts.ValueType.Category"></ChartPrimaryXAxis>
<ChartPrimaryYAxis Title="Sales in Dollar"></ChartPrimaryYAxis>
<ChartTooltipSettings Enable="true"></ChartTooltipSettings>
<ChartSeriesCollection>
<ChartSeries DataSource="@Sales" XName="Month" YName="SalesValue" Type="ChartSeriesType.Column">
</ChartSeries>
</ChartSeriesCollection>
</SfChart>
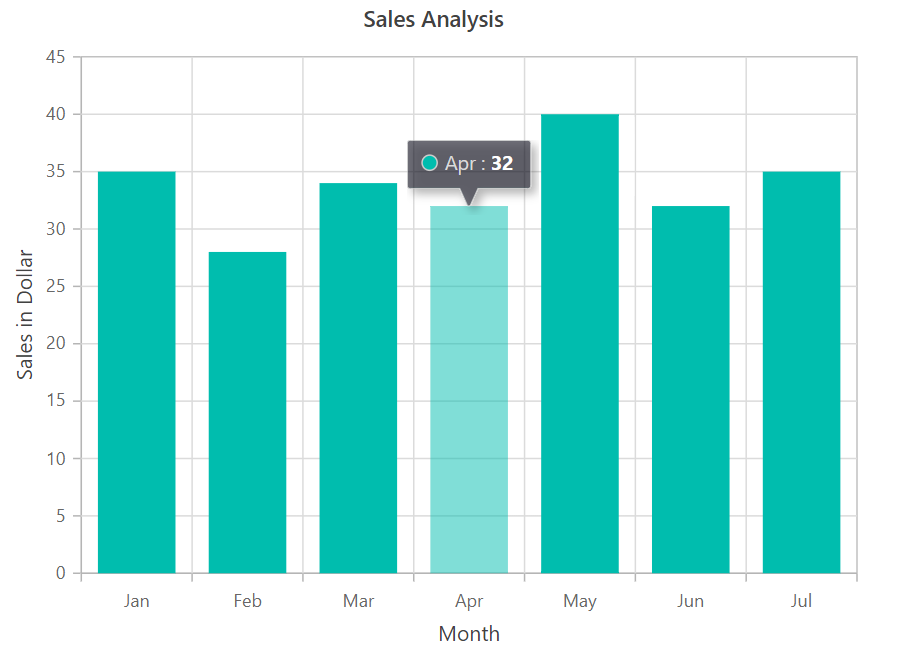
Enable legend
You can use legend for the chart by setting the Visible property to true in ChartLegendSettings. The legend name can be changed by using the Name property in the series.
<SfChart Title="Sales Analysis">
<ChartPrimaryXAxis Title="Month" ValueType="Syncfusion.Blazor.Charts.ValueType.Category"></ChartPrimaryXAxis>
<ChartPrimaryYAxis Title="Sales in Dollar"></ChartPrimaryYAxis>
<ChartLegendSettings Visible="true"></ChartLegendSettings>
<ChartSeriesCollection>
<ChartSeries DataSource="@Sales" Name="Sales" XName="Month" YName="SalesValue" Type="ChartSeriesType.Column">
</ChartSeries>
</ChartSeriesCollection>
</SfChart>
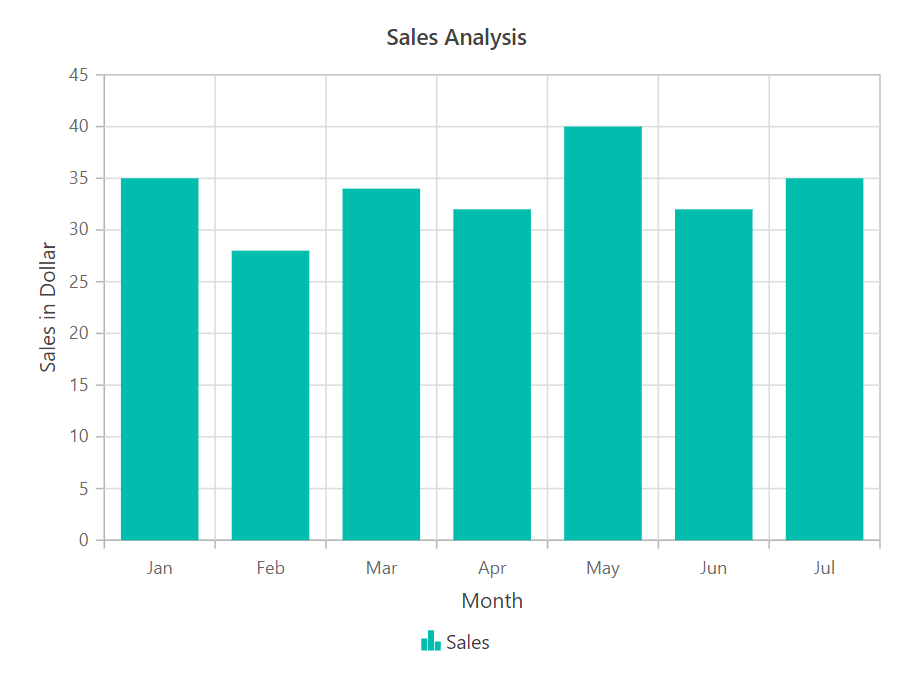
NOTE