Getting Started with Blazor TreeMap in Blazor Web App
5 Dec 20249 minutes to read
This section briefly explains about how to include Blazor TreeMap component in your Blazor Web App using Visual Studio.
Prerequisites
Create a new Blazor Web App
You can create a Blazor Web App using Visual Studio 2022 via Microsoft Templates or the Syncfusion® Blazor Extension.
You need to configure the corresponding Interactive render mode and Interactivity location while creating a Blazor Web Application.
Install Syncfusion® Blazor TreeMap NuGet in the App
To add Blazor TreeMap component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.TreeMap.
If you utilize WebAssembly or Auto
render modes in the Blazor Web App need to be install Syncfusion® Blazor components NuGet packages within the client project.
Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.TreeMap -Version 28.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor
and Syncfusion.Blazor.TreeMap
namespace .
@using Syncfusion.Blazor
@using Syncfusion.Blazor.TreeMap
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor Web App.
If you select an Interactive render mode as WebAssembly
or Auto
, you need to register the Syncfusion® Blazor service in both ~/Program.cs files of your Blazor Web App.
....
using Syncfusion.Blazor;
....
builder.Services.AddSyncfusionBlazor();
....
Add script resources
The script can be accessed from NuGet through Static Web Assets. Include the script reference at the end of the <body>
in the ~/Components/App.razor file as shown below:
<body>
....
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</body>
NOTE
Check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Syncfusion® Blazor TreeMap component
Add the Syncfusion® Blazor TreeMap component in the ~Pages/.razor file. If an interactivity location as Per page/component
in the web app, define a render mode at the top of the ~Pages/.razor
component, as follows:
@* desired render mode define here *@
@rendermode InteractiveAuto
- You can use the DataSource property to load the car sales details in the TreeMap component. Specify a field name from the data source in the WeightValuePath property to calculate the TreeMap item size.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country">
</SfTreeMap>
@code {
public class Country
{
public string Name { get; set; }
public double GDP { get; set; }
}
public List<Country> GrowthReport = new List<Country> {
new Country {Name="United States", GDP=17946 },
new Country {Name="China", GDP=10866 },
new Country {Name="Japan", GDP=4123 },
new Country {Name="Germany", GDP=3355 },
new Country {Name="United Kingdom", GDP=2848 },
new Country {Name="France", GDP=2421 },
new Country {Name="India", GDP=2073 },
new Country {Name="Italy", GDP=1814 },
new Country {Name="Brazil", GDP=1774 },
new Country {Name="Canada", GDP=1550 }
};
}
- Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the application. This will render the Syncfusion® Blazor TreeMap component in your default web browser.
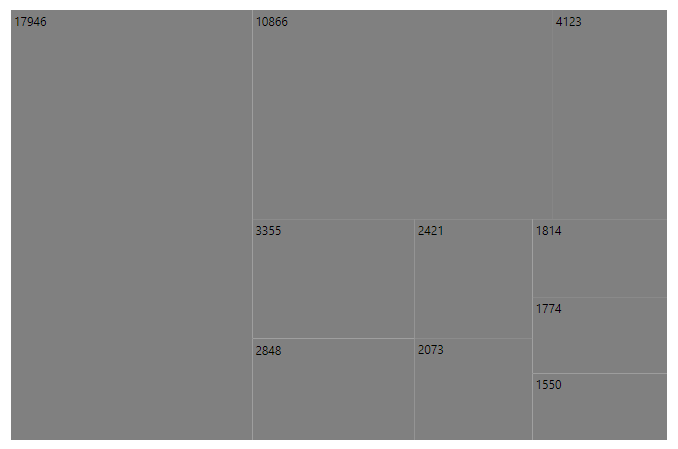
NOTE
Adding labels in Blazor TreeMap items
Add label text to the leaf items in the TreeMap component by setting the field name from data source in the LabelPath property in the TreeMapLeafItemSettings, and it provides information to the user about the leaf items.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country">
<TreeMapLeafItemSettings LabelPath="Name" Fill="lightgray"></TreeMapLeafItemSettings>
</SfTreeMap>
NOTE
Refer to the code block to know about the property value of the GrowthReport.
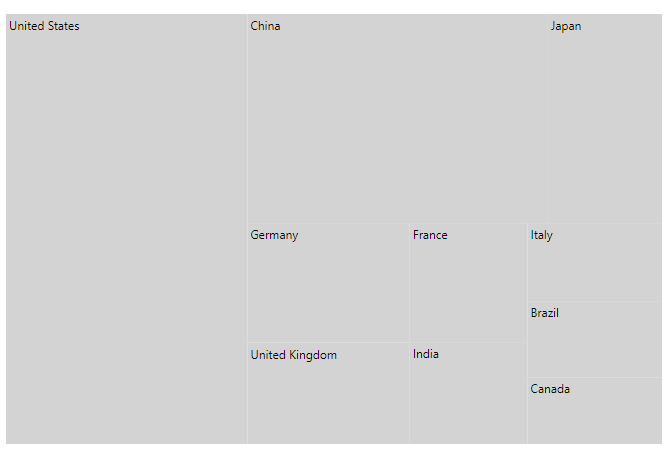
Adding title to Blazor TreeMap
Add a title using the Text property in the TreeMapTitleSettings to provide quick information to the user about the items rendered in the TreeMap.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country">
<TreeMapTitleSettings Text="Top 10 countries by GDP Nominal - 2015"></TreeMapTitleSettings>
<TreeMapLeafItemSettings LabelPath="Name" Fill="lightgray"></TreeMapLeafItemSettings>
</SfTreeMap>
NOTE
Refer to the code block to know about the property value of the GrowthReport.
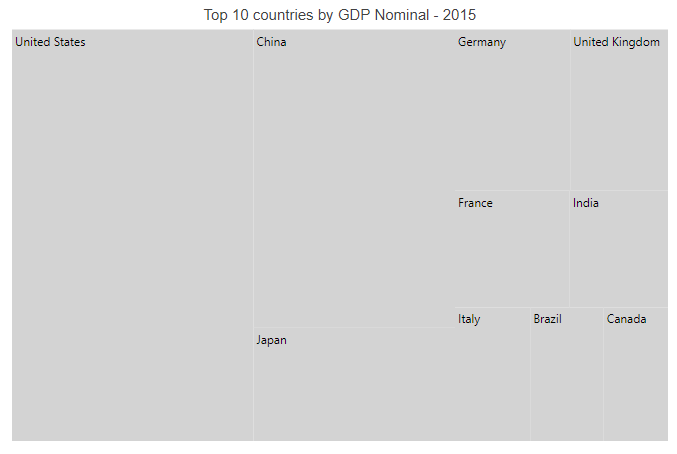
NOTE
Apply color mapping
The color mapping supports customization of item colors based on the underlying value received from the bound data source. Specify the field name from which the values have to be compared for the items in the RangeColorValuePath property in SfTreeMap. Also, specify range value and color in the TreeMapLeafColorMapping. Here, in this example, “Orange” is specified for the range “0 - 3000” and “Green” is specified for the range “3000 - 20000”.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country"
RangeColorValuePath="GDP">
<TreeMapTitleSettings Text="Top 10 countries by GDP Nominal - 2015"></TreeMapTitleSettings>
<TreeMapLeafItemSettings LabelPath="Name" Fill="lightgray">
<TreeMapLeafColorMappings>
<TreeMapLeafColorMapping StartRange="0" EndRange="3000" Color="@(new string[] { "Orange" })"></TreeMapLeafColorMapping>
<TreeMapLeafColorMapping StartRange="3000" EndRange="20000" Color="@(new string[] { "Green" })"></TreeMapLeafColorMapping>
</TreeMapLeafColorMappings>
</TreeMapLeafItemSettings>
</SfTreeMap>
NOTE
Refer to the code block to know about the property value of the GrowthReport.
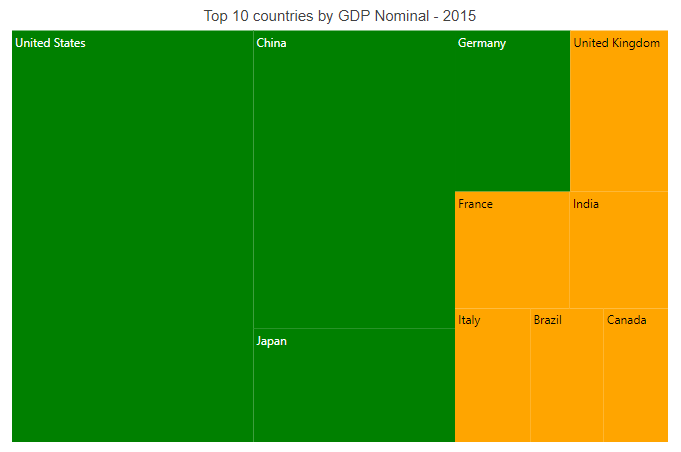
Enable legend
Legend items are used to denote the color mapping categories and show the legend for the TreeMap by setting the Visible property to true in the TreeMapLegendSettings.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country"
RangeColorValuePath="GDP">
<TreeMapTitleSettings Text="Top 10 countries by GDP Nominal - 2015"></TreeMapTitleSettings>
<TreeMapLeafItemSettings LabelPath="Name" Fill="lightgray">
<TreeMapLeafColorMappings>
<TreeMapLeafColorMapping StartRange="0" EndRange="3000" Color="@(new string[] { "Orange" })"></TreeMapLeafColorMapping>
<TreeMapLeafColorMapping StartRange="3000" EndRange="20000" Color="@(new string[] { "Green" })"></TreeMapLeafColorMapping>
</TreeMapLeafColorMappings>
</TreeMapLeafItemSettings>
<TreeMapLegendSettings Visible="true"></TreeMapLegendSettings>
</SfTreeMap>
NOTE
Refer to the code block to know about the property value of the GrowthReport.
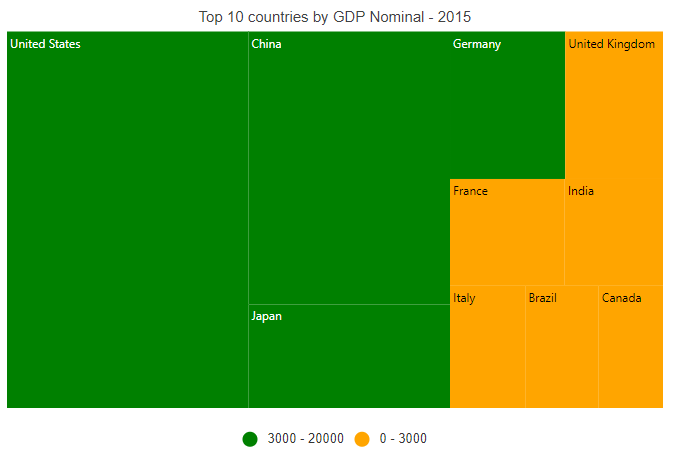
Enable tooltip
When space constraints prevents from displaying information using data labels, the tooltip comes in handy. The tooltip can be enabled by setting the Visible property to true in the TreeMapTooltipSettings.
<SfTreeMap DataSource="GrowthReport"
WeightValuePath="GDP"
TValue="Country"
RangeColorValuePath="GDP">
<TreeMapTitleSettings Text="Top 10 countries by GDP Nominal - 2015"></TreeMapTitleSettings>
<TreeMapLeafItemSettings LabelPath="Name" Fill="lightgray">
<TreeMapLeafColorMappings>
<TreeMapLeafColorMapping StartRange="0" EndRange="3000" Color="@(new string[] { "Orange" })"></TreeMapLeafColorMapping>
<TreeMapLeafColorMapping StartRange="3000" EndRange="20000" Color="@(new string[] { "Green" })"></TreeMapLeafColorMapping>
</TreeMapLeafColorMappings>
</TreeMapLeafItemSettings>
<TreeMapLegendSettings Visible="true"></TreeMapLegendSettings>
<TreeMapTooltipSettings Visible="true"></TreeMapTooltipSettings>
</SfTreeMap>
NOTE
Refer to the code block to know about the property value of the GrowthReport.
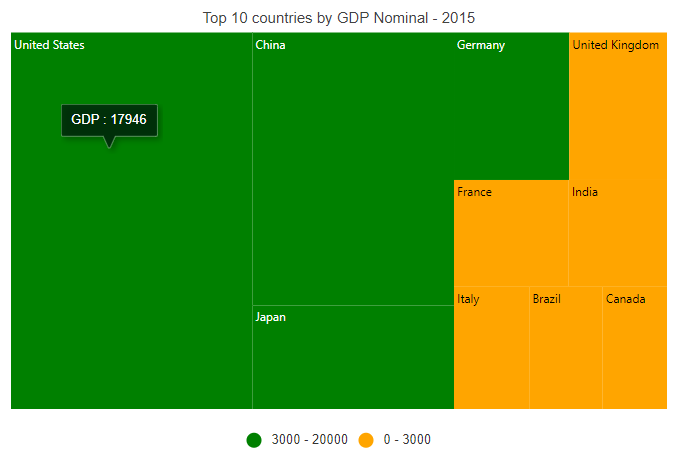
NOTE
You can also explore our Blazor TreeMap example that shows you how to render and configure the treemap.