Getting Started with Blazor Gantt Chart Component
12 Mar 202523 minutes to read
This section briefly explains about how to include Blazor Gantt Chart component in your Blazor WebAssembly App using Visual Studio and Visual Studio Code.
To get started quickly with Blazor Gantt Chart component, check on the following video:
Prerequisites
Create a new Blazor App in Visual Studio
You can create a Blazor WebAssembly App using Visual Studio via Microsoft Templates or the Syncfusion® Blazor Extension.
Install Syncfusion® Blazor Gantt and Themes NuGet in the App
To add Blazor Gantt Chart component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.Gantt and Syncfusion.Blazor.Themes. Alternatively, you can utilize the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.Gantt -Version 29.1.33
Install-Package Syncfusion.Blazor.Themes -Version 29.1.33
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Prerequisites
Create a new Blazor App in Visual Studio Code
You can create a Blazor WebAssembly App using Visual Studio Code via Microsoft Templates or the Syncfusion® Blazor Extension.
Alternatively, you can create a WebAssembly application using the following command in the terminal(Ctrl+`).
dotnet new blazorwasm -o BlazorApp
cd BlazorApp
Install Syncfusion® Blazor Gantt and Themes NuGet in the App
- Press Ctrl+` to open the integrated terminal in Visual Studio Code.
- Ensure you’re in the project root directory where your
.csproj
file is located. - Run the following command to install a Syncfusion.Blazor.Gantt and Syncfusion.Blazor.Themes NuGet package and ensure all dependencies are installed.
dotnet add package Syncfusion.Blazor.Gantt -v 29.1.33
dotnet add package Syncfusion.Blazor.Themes -v 29.1.33
dotnet restore
NOTE
Syncfusion® Blazor components are available in nuget.org. Refer to NuGet packages topic for available NuGet packages list with component details.
Register Syncfusion® Blazor Service
Open ~/_Imports.razor file and import the Syncfusion.Blazor
and Syncfusion.Blazor.Gantt
namespace.
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Gantt
Now, register the Syncfusion® Blazor Service in the ~/Program.cs file of your Blazor WebAssembly App.
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Syncfusion.Blazor;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddSyncfusionBlazor();
await builder.Build().RunAsync();
....
Add stylesheet and script resources
The theme stylesheet and script can be accessed from NuGet through Static Web Assets. Include the stylesheet and script references in the <head>
section of the ~/index.html file.
<head>
....
<link href="_content/Syncfusion.Blazor.Themes/bootstrap5.css" rel="stylesheet" />
<script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script>
</head>
NOTE
Check out the Blazor Themes topic to discover various methods (Static Web Assets, CDN, and CRG) for referencing themes in your Blazor application. Also, check out the Adding Script Reference topic to learn different approaches for adding script references in your Blazor application.
Add Blazor Gantt Chart component
Add the Syncfusion® Blazor Gantt Chart component in the ~/Pages/Index.razor file.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
<GanttColumns>
<GanttColumn Field="TaskId" HeaderText="Task ID"></GanttColumn>
<GanttColumn Field="TaskName" HeaderText="Task Name"></GanttColumn>
<GanttColumn Field="StartDate" HeaderText="Start Date"></GanttColumn>
<GanttColumn Field="Duration" HeaderText="Duration"></GanttColumn>
<GanttColumn Field="Progress" HeaderText="Progress"></GanttColumn>
</GanttColumns>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
Binding Gantt Chart with Data and Mapping Task Fields
Bind data with the Gantt Chart component by using the DataSource property. It accepts the list objects or the DataManager instance.
Additionally, task-related fields from the data source are mapped to the Gantt Chart component using the GanttTaskFields property. This property ensures that the necessary task fields, such as Id, Name, StartDate, EndDate, Duration, and ParentID are properly linked to the corresponding data source fields, allowing the Gantt Chart to render tasks accurately. The columns in the Gantt Chart are automatically rendered based on the properties specified in GanttTaskFields
, ensuring that the necessary columns are displayed to represent the task data.
This following sample shows self-referential data binding in the Gantt Chart by mapping the data source fields to the Id
and ParentID
properties. For more detailed information, refer to the documentation.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
Defining columns
Gantt Chart has an option to define columns as an array. You can manage the order and customize the Gantt Chart columns using the following properties:
- Field: Maps the data source fields to the columns.
- HeaderText: Changes the title of columns.
-
TextAlign: Changes the alignment of columns. By default, columns will be left aligned. To change the columns to right align, set
TextAlign
to right. - Format: Formats the number and date values to standard or custom formats. Here, it is defined for the conversion of numeric values to currency.
- Visible: Show or hide a particular column. By default, columns are visible. Set this property to false to hide the column or true to make it visible.
- Width: To specify the width of the column, which can be defined in pixels or as a percentage of the total column width.
@using Syncfusion.Blazor.Grids
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
<GanttColumns>
<GanttColumn Field="TaskId" HeaderText="Task ID" TextAlign="TextAlign.Right" Width="100"></GanttColumn>
<GanttColumn Field="TaskName" HeaderText="Task Name" Width="250"></GanttColumn>
<GanttColumn Field="StartDate" HeaderText="Start Date" Width="250"></GanttColumn>
<GanttColumn Field="Duration" HeaderText="Duration" Width="250"></GanttColumn>
<GanttColumn Field="Progress" HeaderText="Progress" Format="@NumberFormat" Width="250"></GanttColumn>
</GanttColumns>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
private string NumberFormat = "C";
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
For further details regarding columns, refer here.
Enable editing
The editing feature enables you to edit the tasks in the Gantt Chart component. It can be enabled by using the EditSettings.AllowEditing and EditSettings.AllowTaskbarEditing properties.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
<GanttEditSettings AllowEditing="true" Mode="Syncfusion.Blazor.Gantt.EditMode.Auto" AllowTaskbarEditing="true"></GanttEditSettings>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
NOTE
When the edit mode is set to
Auto
, you can change the cells to editable mode by double-clicking anywhere at the Tree Grid and edit the task details in the edit dialog by double-clicking anywhere at the chart.
You can find the full information regarding Editing from here
Enable filtering
The filtering feature enables you to view the reduced amount of records based on filter criteria. Gantt Chart provides the menu filtering support for each column. It can be enabled by setting the AllowFiltering property to true
. Filtering feature can also be customized using the FilterSettings property.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px" AllowFiltering="true">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
You can find the full information regarding Filtering from here
Enable sorting
The sorting feature enables you to order the records. It can be enabled by setting the AllowSorting property to true
. The sorting feature can be customized using the SortSettings property.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px" AllowSorting="true">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 }
};
return Tasks;
}
}
You can find the full information regarding Sorting from here
Enabling Predecessors or Task Relationships
Predecessor or task dependency in the Gantt Chart component is used to depict the relationship between the tasks.
- Start to Start (SS): You cannot start a task until the dependent task starts.
- Start to Finish (SF): You cannot finish a task until the dependent task finishes.
- Finish to Start (FS): You cannot start a task until the dependent task completes.
- Finish to Finish (FF): You cannot finish a task until the dependent task completes.
You can show the relationship in tasks by using theDependency
property as shown in the following code example.
<SfGantt DataSource="@TaskCollection" Height="450px" Width="700px">
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration" Progress="Progress" ParentID="ParentId" Dependency="Predecessor">
</GanttTaskFields>
</SfGantt>
@code{
private List<TaskData> TaskCollection { get; set; }
protected override void OnInitialized()
{
this.TaskCollection = GetTaskCollection();
}
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime StartDate { get; set; }
public DateTime EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public string Predecessor { get; set; }
public int? ParentId { get; set; }
}
public static List<TaskData> GetTaskCollection()
{
List<TaskData> Tasks = new List<TaskData>() {
new TaskData() { TaskId = 1, TaskName = "Project initiation", StartDate = new DateTime(2022, 04, 05), EndDate = new DateTime(2022, 04, 21), },
new TaskData() { TaskId = 2, TaskName = "Identify Site location", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1 },
new TaskData() { TaskId = 3, TaskName = "Perform soil test", StartDate = new DateTime(2022, 04, 05), Duration = "4", Progress = 50, ParentId = 1, Predecessor = "2" }
};
return Tasks;
}
}
You can find the full information regarding Predecessors from here
Handling exceptions
Exceptions that occur during Gantt actions can be handled without stopping the application. These error messages or exception details can be acquired using the OnActionFailure event.
The argument passed to the OnActionFailure event contains the error details returned from the server.
NOTE
We recommend you bind the
OnActionFailure
event during your application development phase, this helps you to find any exceptions. You can pass these exception details to our support team to get a solution as early as possible.
The following sample code demonstrates notifying user when server-side exception has occurred during data operation,
@using Syncfusion.Blazor
@using Syncfusion.Blazor.Data
@using Syncfusion.Blazor.Gantt
@using Syncfusion.Blazor.Grids
<span class="error">@ErrorDetails</span>
<SfGantt TValue="TaskData" Height="450px" Width="700px">
<SfDataManager Url="https://some.com/invalidUrl" Adaptor="Adaptors.UrlAdaptor"></SfDataManager>
<GanttTaskFields Id="TaskId" Name="TaskName" StartDate="StartDate" EndDate="EndDate" Duration="Duration"
Progress="Progress" ParentID="ParentId">
</GanttTaskFields>
<GanttEvents TValue="TaskData" OnActionFailure="ActionFailure"></GanttEvents>
</SfGantt>
<style>
.error {
color: red;
}
</style>
@code{
private string ErrorDetails = "";
public class TaskData
{
public int TaskId { get; set; }
public string TaskName { get; set; }
public DateTime? StartDate { get; set; }
public DateTime? EndDate { get; set; }
public string Duration { get; set; }
public int Progress { get; set; }
public int? ParentId { get; set; }
}
public void ActionFailure(FailureEventArgs args)
{
this.ErrorDetails = args.Error.Message.ToString();
StateHasChanged();
}
}
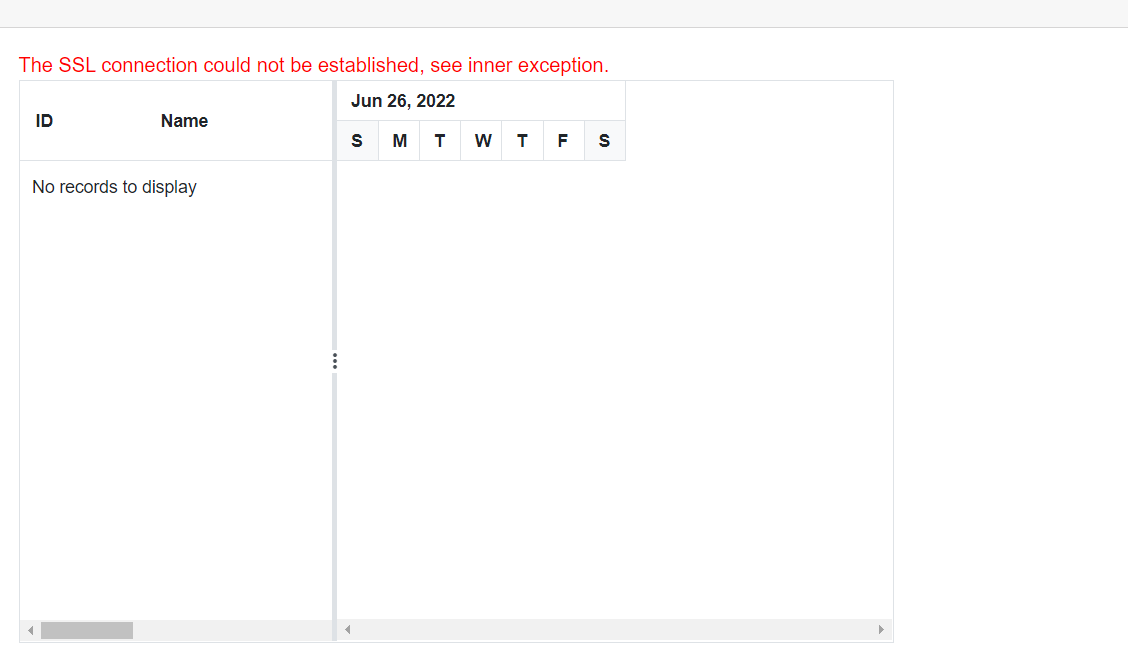
NOTE